QPen¶
- PyQt5.QtGui.QPen
Description¶
The QPen class defines how a QPainter should draw lines and outlines of shapes.
A pen has a style(), width(), brush(), capStyle() and joinStyle().
The pen style defines the line type. The brush is used to fill strokes generated with the pen. Use the QBrush class to specify fill styles. The cap style determines the line end caps that can be drawn using QPainter, while the join style describes how joins between two lines are drawn. The pen width can be specified in both integer (width()) and floating point (widthF()) precision. A line width of zero indicates a cosmetic pen. This means that the pen width is always drawn one pixel wide, independent of the transformation set on the painter.
The various settings can easily be modified using the corresponding setStyle(), setWidth(), setBrush(), setCapStyle() and setJoinStyle() functions (note that the painter’s pen must be reset when altering the pen’s properties).
For example:
# QPainter painter(this);
# QPen pen(Qt::green, 3, Qt::DashDotLine, Qt::RoundCap, Qt::RoundJoin);
# painter.setPen(pen);
which is equivalent to
# QPainter painter(this);
# QPen pen; // creates a default pen
# pen.setStyle(Qt::DashDotLine);
# pen.setWidth(3);
# pen.setBrush(Qt::green);
# pen.setCapStyle(Qt::RoundCap);
# pen.setJoinStyle(Qt::RoundJoin);
# painter.setPen(pen);
The default pen is a solid black brush with 1 width, square cap style (SquareCap), and bevel join style (BevelJoin).
In addition QPen provides the color() and setColor() convenience functions to extract and set the color of the pen’s brush, respectively. Pens may also be compared and streamed.
For more information about painting in general, see the Paint System documentation.
Pen Style¶
Qt provides several built-in styles represented by the PenStyle enum:
Simply use the setStyle() function to convert the pen style to either of the built-in styles, except the CustomDashLine style which we will come back to shortly. Setting the style to NoPen tells the painter to not draw lines or outlines. The default pen style is SolidLine.
Since Qt 4.1 it is also possible to specify a custom dash pattern using the setDashPattern() function which implicitly converts the style of the pen to CustomDashLine. The pattern argument, a QVector, must be specified as an even number of qreal entries where the entries 1, 3, 5… are the dashes and 2, 4, 6… are the spaces. For example, the custom pattern shown above is created using the following code:
# QPen pen;
# QVector<qreal> dashes;
# qreal space = 4;
# dashes << 1 << space << 3 << space << 9 << space
# << 27 << space << 9 << space;
# pen.setDashPattern(dashes);
Note that the dash pattern is specified in units of the pens width, e.g. a dash of length 5 in width 10 is 50 pixels long.
The currently set dash pattern can be retrieved using the dashPattern() function. Use the isSolid() function to determine whether the pen has a solid fill, or not.
Cap Style¶
The cap style defines how the end points of lines are drawn using QPainter. The cap style only apply to wide lines, i.e. when the width is 1 or greater. The PenCapStyle enum provides the following styles:
The SquareCap style is a square line end that covers the end point and extends beyond it by half the line width. The FlatCap style is a square line end that does not cover the end point of the line. And the RoundCap style is a rounded line end covering the end point.
The default is SquareCap.
Whether or not end points are drawn when the pen width is 0 or 1 depends on the cap style. Using SquareCap or RoundCap they are drawn, using FlatCap they are not drawn.
Join Style¶
The join style defines how joins between two connected lines can be drawn using QPainter. The join style only apply to wide lines, i.e. when the width is 1 or greater. The PenJoinStyle enum provides the following styles:
The BevelJoin style fills the triangular notch between the two lines. The MiterJoin style extends the lines to meet at an angle. And the RoundJoin style fills a circular arc between the two lines.
The default is BevelJoin.
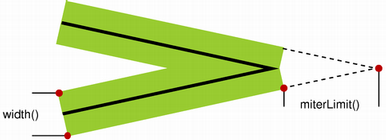
When the MiterJoin style is applied, it is possible to use the setMiterLimit() function to specify how far the miter join can extend from the join point. The miterLimit() is used to reduce artifacts between line joins where the lines are close to parallel.
The miterLimit() must be specified in units of the pens width, e.g. a miter limit of 5 in width 10 is 50 pixels long. The default miter limit is 2, i.e. twice the pen width in pixels.
`The Path Stroking Example <https://doc.qt.io/qt-5/qtwidgets-painting-pathstroke-example.html>`_ The Path Stroking example shows Qt’s built-in dash patterns and shows how custom patterns can be used to extend the range of available patterns. |
See also
Methods¶
- __init__()
Constructs a default black solid line pen with 1 width.
- __init__(PenStyle)
Constructs a black pen with 1 width and the given style.
See also
- __init__(Union[QPen, QColor, GlobalColor, QGradient])
TODO
- __init__(Any)
TODO
- __init__(Union[QBrush, QColor, GlobalColor, QGradient], float, style: PenStyle = SolidLine, cap: PenCapStyle = SquareCap, join: PenJoinStyle = BevelJoin)
TODO
- brush() → QBrush
See also
- capStyle() → PenCapStyle
See also
- color() → QColor
See also
- dashOffset() → float
See also
- dashPattern() → List[float]
See also
- __eq__(Union[QPen, QColor, GlobalColor, QGradient]) → bool
TODO
- isCosmetic() → bool
TODO
- isSolid() → bool
TODO
- joinStyle() → PenJoinStyle
See also
- miterLimit() → float
See also
- __ne__(Union[QPen, QColor, GlobalColor, QGradient]) → bool
TODO
- setBrush(Union[QBrush, QColor, GlobalColor, QGradient])
See also
- setCapStyle(PenCapStyle)
See also
- setColor(Union[QColor, GlobalColor, QGradient])
See also
- setCosmetic(bool)
See also
- setDashOffset(float)
See also
- setDashPattern(Iterable[float])
See also
- setJoinStyle(PenJoinStyle)
See also
- setMiterLimit(float)
See also
- setWidth(int)
See also
- setWidthF(float)
See also
- style() → PenStyle
See also
- swap(QPen)
TODO
- width() → int
See also
- widthF() → float
See also